- This is a quite known issue with arrays/lists in custom Editors. If you checkout EditorGUILayout.PropertyField you will see there are overloads taking a parameter.
- You should not override the OnGUI method in OdinEditorWindows. Instead, if you want to inject custom GUI code, then you override either the DrawEditors method or you can use the OnInspectorGUI attribute on a custom GUI method. If you do have to override the OnGUI method for some reason, then make sure to call base.OnGUI.
- There are a few ways you can do this. You could use the OnInspectorGUI attribute like so, and maybe spice it up with some groups. Public class MyComponent: MonoBehaviour SerializeField, BoxGroup('Split/Some Settings') private int a, b, c; OnInspectorGUI PropertyOrder(-10), HorizontalGroup('Split', 100) private void ShowImage.
I ended up asking over at Unity Answers, and I was quickly given a reliable answer from Bunny83, including a working example of a custom inspector with custom drawing logic.Ultimately, you can draw lines over a custom inspector using OpenGL, I was just doing it wrong. Of particular note, the answer I received made note of additional requirements I had missed.
Unity Pro Tip: Use custom made assets as configuration files
Often times throughout the course of developing a game you end up building some components that need to take in some data through some sort of a configuration file. This could be some parameters for your procedural level generation system, maybe a gesture set for your gesture recognition system, or really any number of things. If you’re developing inside of Unity you probably start this task off by creating a serializable class that is designed as a simple container to hold all the configuration data you are going to need.
But then what? How are you actually going to get your data into that class? Do you create a bunch of XML or JSON files and load them in when the game starts? Maybe you have a public instance of your data class inside of the class that needs the data, and you’re setting everything through the inspector, and then creating a ton of prefabs, one for each of the various configurations.
Well in this post I’m going to show you a simple way to turn those serializable data container classes into custom asset files. This has a number of benefits over using XML or some other external file format. For instance, in general, your file sizes will be much smaller and Unity will handle all the serializing and deserializing for you.
I think this sort of thing is best explained through an example. So I’m going to pretend we’re trying to build a JRPG type dialogue system, like those seen in “Fire Emblem” or “Harvest Moon” and hordes of other JRPGs. You know the type. They have a big text bubble that slides up from the bottom and then a flat picture of the character speaking either on the left or right.
Okay let’s start with creating our simple serializable data container for each individual dialogue speech element as so:
Oninspectorgui
Above we have a couple of enums to describe what characters and screen positions are available, a texture that will be used for displaying the character, a string for what the character is actually saying in the speech bubble, a GUIStyle to style that string with, and maybe a float that we will use later to control how fast the text is displayed back, and an audio clip so we can play back a sound for each speech bubble.
Remember this is just an example so you could use the same techniques for any type of data container like the one above.
Next let’s create our class for a whole dialogue and it will just hold a list of those DialogueElement objects. But instead of making it a plain serializable class we will let it inherit from ScriptableObject. You know, that thing that you always knew was there but were never really sure why? The ScriptableObject class will give us the magic of being able to turn our dialogue class into our own custom asset files.
Now to be able to make custom dialogue assets from that dialog class, you need to download this CustomAssetUtility class and save it somewhere in your project folder. You can download it from this link (right click save as), or if you want you can copy and paste the source from here.
Once you have that installed in your project you can turn that dialog class or any of your classes that inherit from ScriptableObject into a custom assets super easily with just a couple lines.
Just make a new class/file called DialogueAsset.cs and make sure it’s inside an Editor folder. Then inside that class create a menu item for your new asset that just calls CreateAsset from the downloaded utility class like so:
Now when you go to Assets -> Create in the menubar or click the create button in your project view you’ll see an option to create a new dialogue.
And when you click on the newly created asset file the inspector view will show your list of DialogueElements and all their public variables. Just like it would’ve if you had created an instance of DialogueElement inside of a MonoBehavior. But unlike instanced DialogueElements, since these are in their own asset file any changes you make to them will remain persistent throughout edit time and runtime. So if you change some of the values here while the game is running in preview mode the changes will stay even after you stop running the game. This same technique is essentially how GUISkin and a number of Unity’s other built-in assets work.
Now to get access to the data from within another class, say the class you’re writing to actually display the dialogue on-screen, just make a public instance of the dialogue.
Then just assign it through the inspector. Either by clicking the little circle and selecting it from the list that comes up or just dragging and dropping one of your newly created asset files in the open slot.
If you’re feeling ambitious, or just want to take the extra step you can go on to write a custom inspector class to configure how your assets are going to be displayed within the inspector view. If so, you’re going to want to create a new class/file and call it something like DialogueEditor.cs and make sure it is also placed within an Editor folder. Then you’ll want to start off writing that class with something like this:
Then fill in the OnInspectorGUI function with all the code to display the options for the editing your asset that you want. This can be great for cleaning up and simplifying what is displayed in the inspector view, or hiding away options that you don’t want edited. It’s also great for working on teams. It’s really nice to be able to go to your less techno-savvy team members, the script writer for instance, and say “Look it’s really easy. Just click create dialogue and fill everything in.” Here’s a screenshot of what you could do with creating a custom inspector for this example dialogue class.
Oh but there is one small caveat. If you do write a custom inspector class for editing your asset files, or if you change any of the values within one of your custom assets from another MonoBehavior during runtime. You will need to call Unity’s SetDirty method on the object that you changed like so:
This is so that Unity will know that the contents of the object have been changed and needs to be re-serialized and saved to disk.
So there you have it. How to make your own custom assets and use them as configuration files.
If you found this tutorial helpful please let me know in the comments, and as always you can stay updated with all my tutorials and plug-ins by following me on twitter.
Author: Mift (mift)
Note: An updated version of this script package (which only calls a property's setter when the value has actually changed) can be found here: ExposePropertiesInInspector_SetOnlyWhenChanged
|
Description
Unity Override Void
The code here provided is intended for those that want to hold their variables private and let them access only through get/set accessors, so further processing becomes possible.
The Sausage
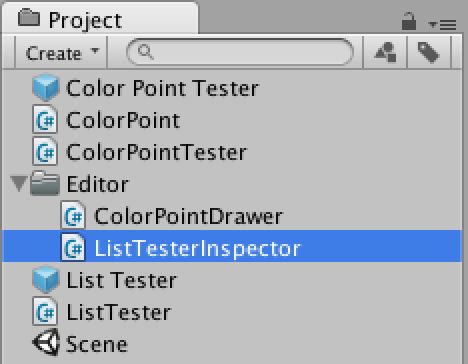
Create a new c# Script named 'ExposePropertyAttribute' and paste following code :
C# - ExposePropertyAttribute.cs
The magic
Now create another script in the 'Assets/Editor' folder named 'ExposeProperties' and paste following code:
C# - ExposeProperties.cs
Note

As you probably have seen in the code above, only following types are currently exposed, but it should be easy enough to implement other ones:
Example component
Now create your type, i.e. MyType. Properties MUST have both getter and setter accessors AND [ExposeProperty] attribute set otherwise the property will not be shown.
In order for the property to maintain values when you hit play, you must add [SerializeField] to the field that the property is changing. Unfortunately, this exposes the field to the Editor (editing this value does not call the property getter/setter), so you have to explicitly hide it with [HideInInspector].
C# - MyType.cs
The custom editor
Finally, create a custom editor Script in 'Assets/Editor' for the type which properties you want to see exposed. I.e.:
C# - MyTypeEditor.cs
Conclusion
Well, thats pretty much the basic system, Im sure some ppl that might touch this 'sauce code' will be able to make it better ( and prettier ). It does what I need it for, so Im not gonna improve it.
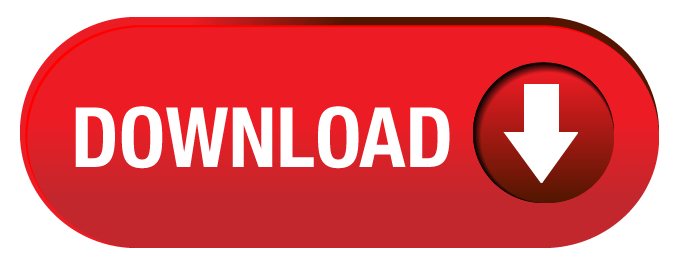